activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/rl" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="16dp" tools:context=".MainActivity" android:background="#e1e6db" > <EditText android:id="@+id/et" android:layout_width="450dp" android:layout_height="wrap_content" android:inputType="textMultiLine" android:maxLines="3" android:minLines="3" android:background="#b7afaf" android:padding="5dp" android:layout_margin="10dp" /> <!-- android:gravity Specifies how to align the text by the view's x- and/or y-axis when the text is smaller than the view. Must be one or more (separated by '|') of the following constant values. top : Push object to the top of its container, not changing its size. bottom : Push object to the bottom of its container, not changing its size. left : Push object to the left of its container, not changing its size. right : Push object to the right of its container, not changing its size. center_vertical, fill_vertical, center_horizontal, fill_horizontal, center, fill, clip_vertical, clip_horizontal, start and end. --> <EditText android:id="@+id/et_second" android:layout_width="450dp" android:layout_height="wrap_content" android:inputType="textMultiLine" android:maxLines="3" android:minLines="3" android:background="#378aff" android:padding="5dp" android:layout_margin="10dp" android:layout_below="@id/et" android:gravity="top" /> <EditText android:id="@+id/et_third" android:layout_width="450dp" android:layout_height="wrap_content" android:inputType="textMultiLine" android:maxLines="3" android:minLines="3" android:background="#66be7e" android:padding="5dp" android:layout_margin="10dp" android:layout_below="@id/et_second" /> </RelativeLayout>
MainActivity.java
package com.cfsuman.me.androidcodesnippets; import android.app.Activity; import android.content.Context; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.Gravity; import android.widget.EditText; import android.widget.RelativeLayout; public class MainActivity extends AppCompatActivity { private Context mContext; private Activity mActivity; private RelativeLayout mRelativeLayout; private EditText mEditText; private EditText mEditTextSecond; private EditText mEditTextThird; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Get the application context mContext = getApplicationContext(); // Get the activity mActivity = MainActivity.this; // Get the widgets reference from XML layout mRelativeLayout = (RelativeLayout) findViewById(R.id.rl); mEditText = (EditText) findViewById(R.id.et); mEditTextSecond = (EditText) findViewById(R.id.et_second); mEditTextThird = (EditText) findViewById(R.id.et_third); /* public void setGravity (int gravity) Sets the horizontal alignment of the text and the vertical gravity that will be used when there is extra space in the TextView beyond what is required for the text itself. Parameters gravity : int */ // Set the vertical alignment programmatically for third EditText // Specify the vertical alignment/gravity to bottom mEditTextThird.setGravity(Gravity.BOTTOM); } }
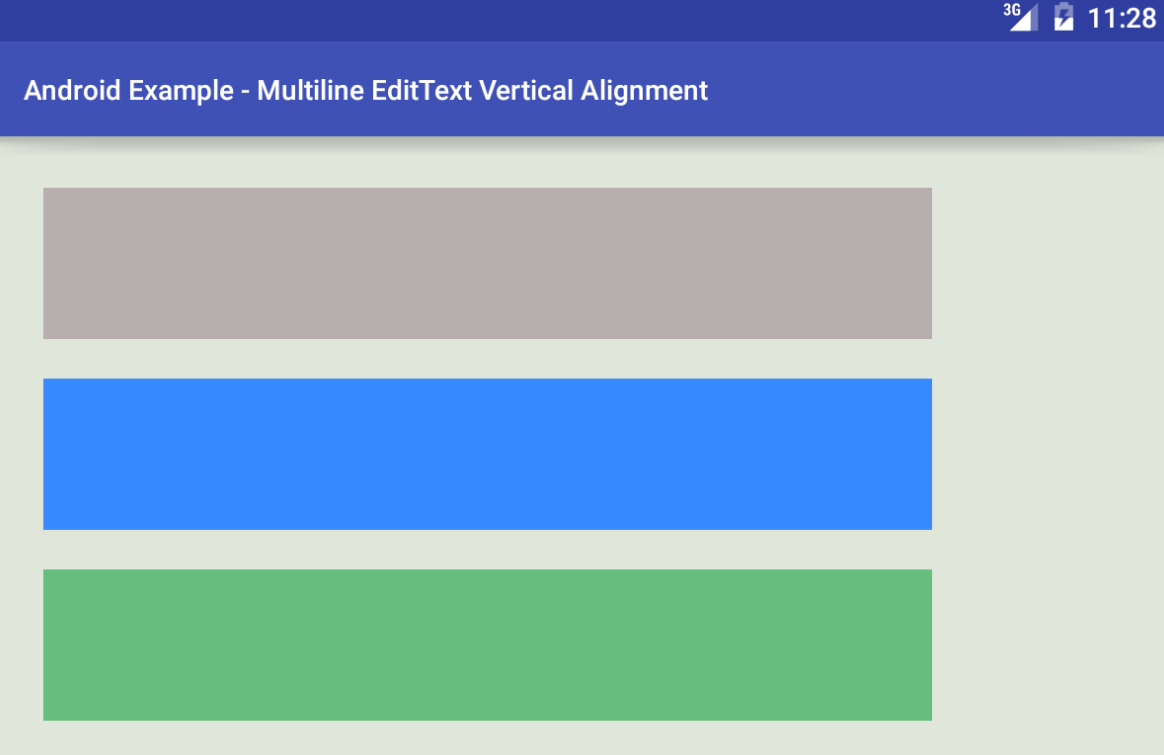

Komentar
Posting Komentar