activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/rl" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="10dp" tools:context=".MainActivity" android:background="#fcfdfb" > <Button android:id="@+id/btn_block_images" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Block Image Loading" /> <WebView android:id="@+id/web_view" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_below="@id/btn_block_images" /> </RelativeLayout>
MainActivity.java
package com.cfsuman.me.androidcodesnippets; import android.app.Activity; import android.content.Context; import android.graphics.Bitmap; import android.graphics.Color; import android.graphics.drawable.ColorDrawable; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.view.Window; import android.webkit.WebChromeClient; import android.webkit.WebView; import android.webkit.WebViewClient; import android.widget.Button; import android.widget.RelativeLayout; public class MainActivity extends AppCompatActivity { private Context mContext; private Activity mActivity; private RelativeLayout mRelativeLayout; private WebView mWebView; private Button mButtonBlockImages; private String mUrl="http://android.oms.apps.opera.com/en_us/"; @Override protected void onCreate(Bundle savedInstanceState) { // Request window feature action kafe requestWindowFeature(Window.FEATURE_ACTION_BAR); super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Get the application context mContext = getApplicationContext(); // Get the activity mActivity = MainActivity.this; // Change the action kafe color getSupportActionBar().setBackgroundDrawable( new ColorDrawable(Color.parseColor("#FF508FDC")) ); // Get the widgets reference from XML layout mRelativeLayout = (RelativeLayout) findViewById(R.id.rl); mWebView = (WebView) findViewById(R.id.web_view); mButtonBlockImages = (Button) findViewById(R.id.btn_block_images); // Request to render the web page renderWebPage(mUrl); // Set a click listener for block image button mButtonBlockImages.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { /* public WebSettings getSettings () Gets the WebSettings object used to control the settings for this WebView. Returns a WebSettings object that can be used to control this WebView's settings */ /* WebSettings Manages settings state for a WebView. When a WebView is first created, it obtains a set of default settings. These default settings will be returned from any getter call. A WebSettings object obtained from WebView.getSettings() is tied to the life of the WebView. If a WebView has been destroyed, any method call on WebSettings will throw an IllegalStateException. */ /* public abstract void setBlockNetworkImage (boolean flag) Sets whether the WebView should not load image resources from the network (resources accessed via http and https URI schemes). Note that this method has no effect unless getLoadsImagesAutomatically() returns true. Also note that disabling all network loads using setBlockNetworkLoads(boolean) will also prevent network images from loading, even if this flag is set to false. When the value of this setting is changed from true to false, network images resources referenced by content currently displayed by the WebView are fetched automatically. The default is false. Parameters flag : whether the WebView should not load image resources from the network */ // Block images to load in web view mWebView.getSettings().setBlockNetworkImage(true); /* public abstract void setLoadsImagesAutomatically (boolean flag) Sets whether the WebView should load image resources. Note that this method controls loading of all images, including those embedded using the data URI scheme. Use setBlockNetworkImage(boolean) to control loading only of images specified using network URI schemes. Note that if the value of this setting is changed from false to true, all images resources referenced by content currently displayed by the WebView are loaded automatically. The default is true. Parameters flag : whether the WebView should load image resources */ // Block to loading images automatically mWebView.getSettings().setLoadsImagesAutomatically(false); // Re-render the current page in web view renderWebPage(mUrl); } }); } // Custom method to render a web page protected void renderWebPage(String urlToRender) { mWebView.setWebViewClient(new WebViewClient() { @Override public void onPageStarted(WebView view, String url, Bitmap favicon) { // Do something on page loading started mUrl = url; } @Override public void onPageFinished(WebView view, String url) { // Do something when page loading finished mUrl = url; } }); mWebView.setWebChromeClient(new WebChromeClient() { public void onProgressChanged(WebView view, int newProgress) { } }); // Enable the javascript mWebView.getSettings().setJavaScriptEnabled(true); // Render the web page mWebView.loadUrl(urlToRender); } }

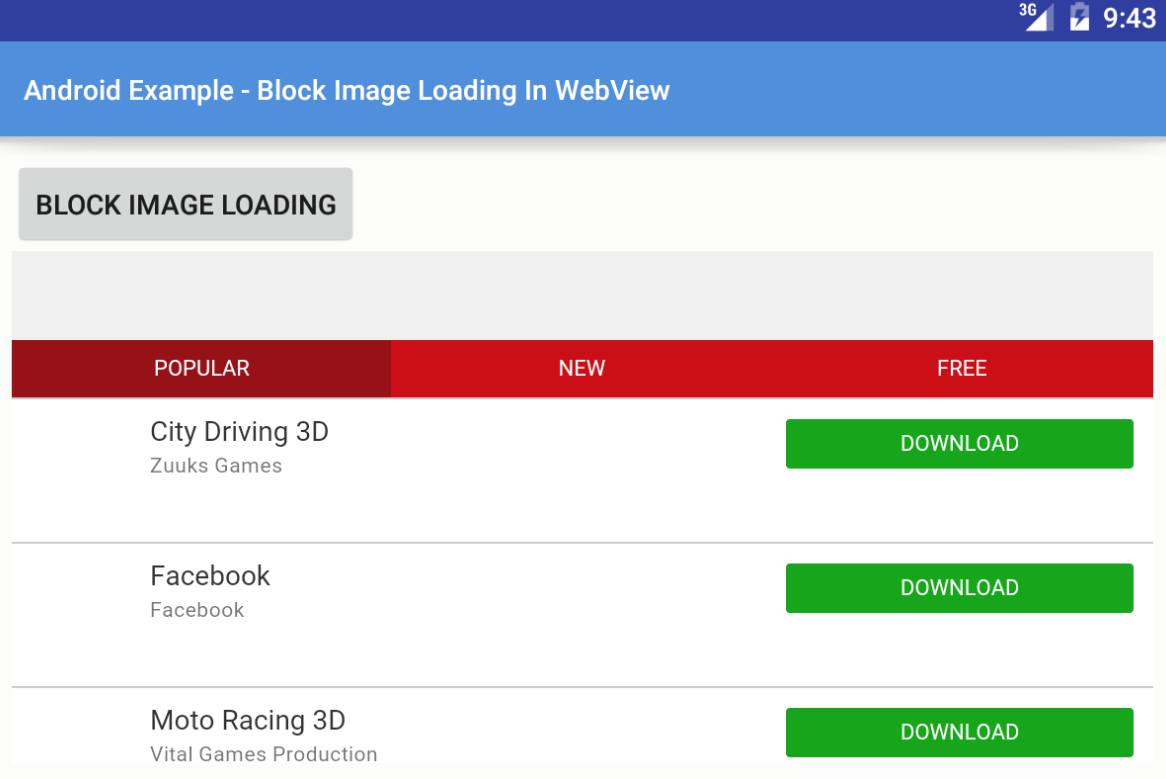
Komentar
Posting Komentar